JavaScript is the linchpin of modern web development, the driving force behind dynamic and interactive web applications. While developers frequently engage with JavaScript on the surface, understanding its inner workings is a fundamental step toward mastering this versatile language. In this comprehensive guide, we'll delve into the intricate mechanics of JavaScript, shedding light on the core concepts that power its functionality.
The JavaScript Engine
The JavaScript Engine: The Heart of Execution
At the heart of JavaScript lies the JavaScript engine, responsible for parsing, interpreting, and executing your code. Google's V8 engine, which powers Chrome, is perhaps the most renowned, while Firefox employs SpiderMonkey, and Edge uses Chakra.
Two Phases: Compilation and Execution
JavaScript isn't a pure interpreted language; it undergoes a two-step process before execution:
Compilation: This phase is also called as memory creation phase or simply creation phase. In this phase, the JavaScript engine parses your code and compiles it into an executable format. It also performs syntax error checks and code optimizations. During this phase, all the variables defined in the code are initialized with ‘undefined’ and functions are stored as function definition. Also, global object is created and is assigned to ‘this’.
Execution: Following successful compilation, the engine proceeds to execute the code line by line, managing variables, scope, and memory during the process. For each function call, a new Execution context is created, and both the phases are executed again for that function.
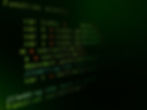
JavaScript Runtime Environment
The Global Object
In any JavaScript runtime environment, there's always a global object, commonly known as window in web browsers. This global object serves as the root for all other objects and variables, representing the environment in which your JavaScript code operates.
Execution Context
Execution context is a pivotal concept in JavaScript, visualized as the 'box' where your code is evaluated and executed. Each function call creates a new execution context, and these contexts can be nested within one another.
There are 2 types of Execution Contexts
Global Execution Context: The initial execution context that envelops your entire JavaScript program.
Function Execution Context: Every function call spawns a new execution context, containing the function's code and its local variables.
The Call Stack
The call stack, a fundamental data structure, keeps track of execution contexts. When a function is called, a new execution context is pushed onto the stack, and when the function returns, the context is popped off the stack. It follows LIFO (Last In First Out) technique.
Variables and Scope
Variable Declarations
JavaScript provides various ways to declare variables, including var, let, and const. The choice of declaration influences variable scope:
Global Scope: Variables declared outside of functions reside in the global scope, making them accessible from any part of your code.
Function Scope: Variables declared within a function using var are function-scoped, limiting their accessibility to that function. But due to Hoisting, they can still be accessible in global scope.
Block Scope: Variables declared within blocks using let and const are block-scoped, only accessible within that block.
Hoisting
JavaScript incorporates the concept of hoisting, where variable and function declarations are moved to the top of their containing scope during compilation. Declarations are hoisted, but assignments are not.
Closures and Lexical Scope
Closures
Closures are a potent and somewhat mystical aspect of JavaScript. They come into play when a function is defined within another function and retains access to the parent function's scope, even after the parent function completes its execution.
Lexical Scope
Lexical scope is the mechanism by which JavaScript determines variable scope. It depends on the physical location of a variable's declaration in the code. This feature allows functions to "capture" variables from their containing scopes, resulting in the creation of closures.
JavaScript Performance Optimization
Enhancing JavaScript Code Performance
Optimizing JavaScript code is critical for web developers. Consider these techniques to enhance the efficiency of your code:
Minification: Reduce the size of your code by eliminating whitespace and shortening variable names.
Caching: Store frequently used data or results to minimize redundant computations.
Event Delegation: Reduce the number of event listeners through event delegation.
JavaScript Engines and JIT Compilation
Modern JavaScript engines utilize Just-In-Time (JIT) compilation to optimize code execution. They compile JavaScript into machine code, significantly boosting its speed. For instance, the V8 engine adopts a two-tiered compilation system for this purpose.
Conclusion: JavaScript Inner Workings Guide
In this comprehensive guide, we've delved into the inner workings of JavaScript, exploring the JavaScript engine, runtime environment, variables, scope, closures, performance optimization, and memory management. A solid understanding of these core concepts will empower you to become a more proficient JavaScript developer, enabling you to write code that is efficient, maintainable, and performant.
JavaScript is a dynamic and ever-evolving language, and staying current with its advancements is essential for any developer. By grasping the foundational principles of how JavaScript operates beneath the surface, you'll be better equipped to harness its capabilities and create web applications that not only meet but exceed the expectations of today's demanding users.
As you continue to explore, experiment, and learn, you will unlock the full potential of JavaScript in your web development journey. Understanding the inner workings of JavaScript is your key to building a successful and thriving career in the ever-expanding world of web development.