Introduction to JavaScript Promises
JavaScript, as one of the most widely used programming languages, plays a pivotal role in web development. It allows developers to create interactive and dynamic web applications.
However, handling asynchronous operations in JavaScript has always been a challenge. This is where Promises come into the picture, making asynchronous programming more manageable and comprehensible.
Promises are a fundamental feature of JavaScript, introduced to address the notorious "callback hell" and provide a cleaner way to handle asynchronous operations. They enable you to write asynchronous code that is more readable, maintainable, and easier to reason about.
In this article, we will delve into the world of JavaScript Promises, exploring how they work, creating and chaining them, handling errors, and finally, understanding their significance in modern web development.
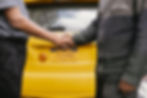
How JavaScript Promises Work
Before diving into the nitty-gritty of Promises, it's essential to understand the problem they solve. JavaScript is single-threaded and non-blocking, meaning it can perform tasks concurrently without waiting for one to finish before moving on to the next. This is particularly useful when dealing with I/O operations like fetching data from a server, reading files, or handling user interactions.
However, handling the results of these asynchronous operations can be tricky. Traditional callback functions are prone to nesting, leading to callback hell or pyramid of doom. Promises were introduced to mitigate this issue and provide a more structured approach to handle asynchronous code.
At its core, a Promise represents a value that might not be available yet but will be at some point in the future. A Promise has three states:
Pending: Initial state when the Promise is created and hasn't resolved or rejected yet.
Fulfilled (Resolved): The operation completed successfully, and the Promise holds the result.
Rejected: The operation encountered an error, and the Promise holds the reason for failure.
Promises are objects that have two primary functions, then() and catch(), which allow you to define what to do when a Promise resolves or rejects. They make asynchronous code more linear and easier to follow.
Creating JavaScript Promises
Creating a Promise is quite straightforward. You can use the Promise constructor to create a new Promise instance. The constructor takes one argument, a function, which has two parameters: resolve and reject. These parameters are functions you call when the Promise should be resolved or rejected.
Here's an example of creating a simple Promise that simulates fetching data from a server:
const fetchData = new Promise((resolve, reject) => {
setTimeout(() => {
const data = { message: "Data fetched successfully" };
resolve(data); // Resolve the Promise with data
}, 2000); // Simulate a 2-second delay
});
fetchData
.then((data) => {
console.log(data.message);
})
.catch((error) => {
console.error("Error:", error);
});
In this example, fetchData is a Promise that resolves after a 2-second delay. When the Promise resolves, the then() block is executed, and we log the message to the console. If an error occurs, the catch() block is executed.
Chaining JavaScript Promises
One of the key benefits of Promises is their ability to chain operations. You can create a sequence of asynchronous operations, where the output of one operation becomes the input for the next. This results in cleaner and more structured code.
Consider a scenario where you want to fetch user details and then fetch their recent orders, which depends on the user data. You can achieve this using Promise chaining:
function fetchUserDetails(userId) {
return new Promise((resolve, reject) => {
// Simulate fetching user details
setTimeout(() => {
const userDetails = { id: userId, name: "John Doe" };
resolve(userDetails);
}, 1000);
});
}
function fetchUserOrders(user) {
return new Promise((resolve, reject) => {
// Simulate fetching user orders based on user details
setTimeout(() => {
const orders = ["Order #1", "Order #2"];
resolve(orders);
}, 1500);
});
}
fetchUserDetails(123)
.then((user) => {
return fetchUserOrders(user);
})
.then((orders) => {
console.log("User Orders:", orders);
})
.catch((error) => {
console.error("Error:", error);
});
In this example, we first fetch user details and then use the user details to fetch their orders. The .then() method is used to chain these Promises, making the code more elegant and easier to read.
Handling Errors with JavaScript Promises
Handling errors in asynchronous code can be tricky but Promises provide an elegant way to deal with them. When an error occurs within a Promise, you can catch it using the catch() method, which is similar to a try-catch block in synchronous code.
Here's an example of handling errors with Promises:
function performRiskyOperation() {
return new Promise((resolve, reject) => {
const isSuccessful = Math.random() < 0.5; // Simulate a risky operation
if (isSuccessful) {
resolve("Operation successful");
} else {
reject("Operation failed");
}
});
}
performRiskyOperation()
.then((result) => {
console.log(result);
})
.catch((error) => {
console.error("Error:", error);
});
In this example, performRiskyOperation simulates a risky operation that may fail. If the operation fails, the Promise is rejected, and the error is caught by the catch() block.
Additionally, you can handle errors in a more centralized manner by using Promise.all() or Promise.race() to work with multiple Promises simultaneously. Promise.all() resolves when all the Promises in an array have resolved and rejects if any of them rejects. On the other hand, Promise.race() resolves or rejects as soon as one of the Promises resolves or rejects.
Conclusion
In conclusion, JavaScript Promises have revolutionized the way we handle asynchronous operations in web development. They provide a structured and elegant approach to managing asynchronous code, mitigating the callback hell problem, and making it more readable and maintainable.
Promises are the foundation of modern JavaScript, enabling you to create robust, error-handling asynchronous workflows. They have become even more critical with the widespread adoption of async/await, which is built on top of Promises.
As you continue to explore the world of web development, mastering Promises will be essential for building efficient and responsive applications. Whether you're fetching data from a server, handling user interactions, or performing any other asynchronous task, Promises are your reliable companions in the journey of JavaScript development.